"Exploring the Essential Libraries of Python: Tools for Every Developer"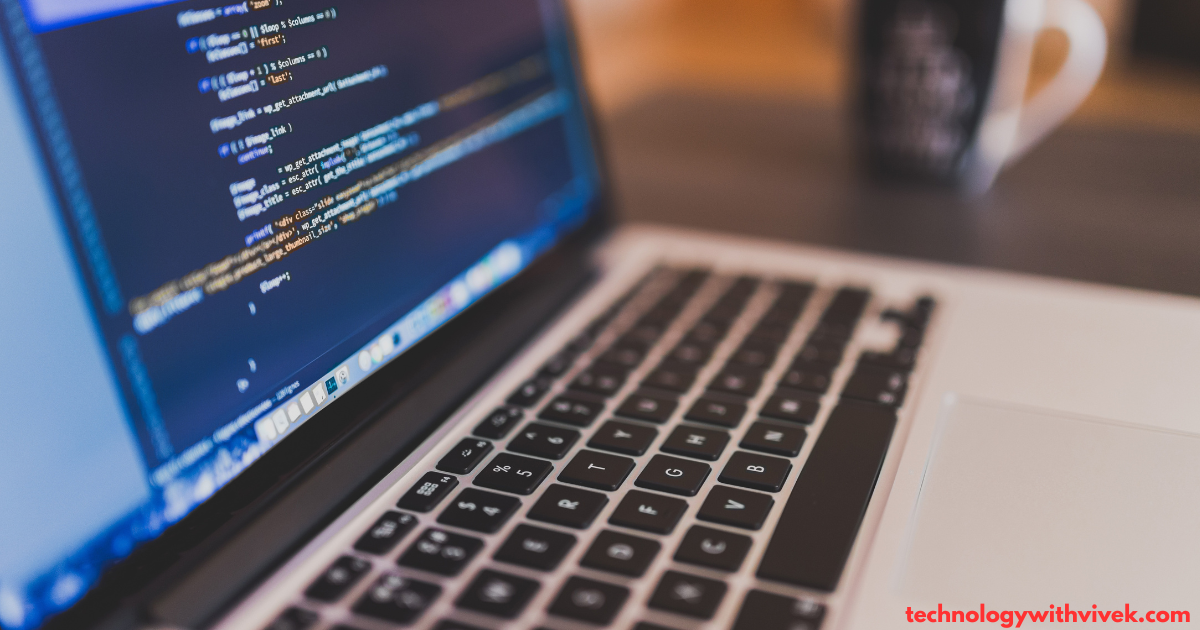.png)
What is NumPy Library in Python?
NumPy, short for Numerical Python, is an open-source library in Python designed for scientific computing. It provides support for large, multi-dimensional arrays and matrices, along with a collection of mathematical functions to operate on these arrays. NumPy is a core library in the scientific Python ecosystem and serves as the foundation for libraries like pandas, SciPy, and TensorFlow.
Key Features of NumPy:
1. Efficient Multidimensional Array Objects: NumPy provides `ndarray`, a versatile and efficient array object, which can store elements of the same data type.
2. Mathematical Operations: NumPy includes a wide range of mathematical functions such as linear algebra, statistics, and more.
3. Broadcasting: NumPy supports broadcasting, allowing operations on arrays of different shapes in a way that they match automatically.
4. Integration with Other Libraries: NumPy integrates seamlessly with libraries like pandas, matplotlib, and more.
5. High Performance: NumPy's functions are implemented in C, making them faster than regular Python lists.
Getting Started with NumPy
To install NumPy, use `pip`:
pip install numpy
Once installed, you can import it into your Python script using:
import numpy as np
Now, let’s look at some basic operations with NumPy.
Example 1: Creating Arrays
The fundamental object in NumPy is the `ndarray`. Here’s how you can create and manipulate arrays:
import numpy as np
# 1D array
arr_1d = np.array([1, 2, 3, 4, 5])
print("1D Array:", arr_1d)
# 2D array
arr_2d = np.array([[1, 2, 3], [4, 5, 6]])
print("\n2D Array:\n", arr_2d)
# Check the dimensions of the array
print("\nArray Dimensions:", arr_2d.ndim)
Output:
1D Array: [1 2 3 4 5]
2D Array:
[[1 2 3]
[4 5 6]]
Array Dimensions: 2
Example 2: Array Operations
NumPy makes it easy to perform element-wise operations on arrays:
import numpy as np
# Creating two arrays
arr1 = np.array([1, 2, 3])
arr2 = np.array([4, 5, 6])
# Element-wise addition
arr_sum = arr1 + arr2
print("Sum of arrays:", arr_sum)
# Element-wise multiplication
arr_product = arr1 * arr2
print("Product of arrays:", arr_product)
# Broadcasting: Adding scalar to array
arr_broadcast = arr1 + 5
print("Broadcasted array:", arr_broadcast)
Output:
Sum of arrays: [5 7 9]
Product of arrays: [ 4 10 18]
Broadcasted array: [6 7 8]
Example 3: Array Slicing and Indexing
You can slice and index NumPy arrays just like Python lists but with additional flexibility:
import numpy as np
arr = np.array([[1, 2, 3], [4, 5, 6], [7, 8, 9]])
# Accessing a specific element
element = arr[1, 2] # Element at 2nd row, 3rd column
print("Element at [1,2]:", element)
# Slicing a subarray
subarray = arr[0:2, 1:3] # First two rows, last two columns
print("\nSubarray:\n", subarray)
Output:
Element at [1,2]: 6
Subarray:
[[2 3]
[5 6]]
Example 4: Mathematical Functions
NumPy comes with numerous built-in mathematical functions that can be applied to arrays:
import numpy as np
arr = np.array([1, 2, 3, 4, 5])
# Calculate square root
sqrt_arr = np.sqrt(arr)
print("Square Root:", sqrt_arr)
# Calculate exponential
exp_arr = np.exp(arr)
print("Exponential:", exp_arr)
# Calculate mean and sum
mean_value = np.mean(arr)
sum_value = np.sum(arr)
print("Mean:", mean_value)
print("Sum:", sum_value)
Output:
Square Root: [1. 1.41421356 1.73205081 2. 2.23606798]
Exponential: [ 2.71828183 7.3890561 20.08553692 54.59815003 148.4131591 ]
Mean: 3.0
Sum: 15
Example 5: Reshaping and Transposing Arrays
You can reshape and transpose arrays to fit your desired structure:
import numpy as np
arr = np.array([[1, 2, 3], [4, 5, 6], [7, 8, 9]])
# Reshape into a 1D array
reshaped_arr = arr.reshape(9)
print("Reshaped Array:", reshaped_arr)
# Transpose the array (rows become columns and vice versa)
transposed_arr = arr.T
print("\nTransposed Array:\n", transposed_arr)
Output:
Reshaped Array: [1 2 3 4 5 6 7 8 9]
Transposed Array:
[[1 4 7]
[2 5 8]
[3 6 9]]
Conclusion
NumPy is a powerful and versatile library that simplifies working with arrays, making it a go-to for scientific computing in Python. With efficient array operations, mathematical functions, and seamless integration with other libraries, NumPy is essential for anyone working with data science, machine learning, or engineering computations.
Understanding NumPy is the first step toward becoming proficient in Python-based scientific computing. Start practicing with arrays and gradually explore advanced features such as broadcasting, linear algebra operations, and more
What is Pandas Library in Python?
Pandas is an open-source data analysis and manipulation library for Python. It provides data structures and functions needed to work with structured data seamlessly. Whether you're handling time series, numerical tables, or categorical data, Pandas simplifies the process by offering powerful and flexible tools to clean, analyze, and manipulate data.
The name "Pandas" is derived from "Panel Data", which refers to multidimensional structured data sets. Built on top of the NumPy library, Pandas enables easy handling of large data sets with highly optimized performance.
Key Features of Pandas:
1. Data Structures:
- Series: A one-dimensional labeled array capable of holding any data type (similar to a column in a spreadsheet).
- DataFrame: A two-dimensional labeled data structure with columns of potentially different types (similar to a table or a spreadsheet).
2. Data Manipulation: Tools to filter, slice, aggregate, and group data easily.
3. Handling Missing Data: Pandas provide intelligent ways to handle missing or null data.
4. Data Import and Export: Easy methods to read and write data from various formats such as CSV, Excel, SQL, JSON, and more.
5. Powerful Data Cleaning: Pandas allows you to clean and prepare data in just a few lines of code.
6. Time-Series Functionality: Built-in support for handling time-series data efficiently.
Getting Started with Pandas
To install Pandas, use the following command:
pip install pandas
You can import Pandas in your Python script using:
import pandas as pd
Let’s look at some common operations in Pandas with example code.
Example 1: Creating a DataFrame
The primary data structure in Pandas is the `DataFrame`. It is similar to a table or spreadsheet with rows and columns.
import pandas as pd
# Creating a DataFrame from a dictionary
data = {
'Name': ['John', 'Anna', 'Peter', 'Linda'],
'Age': [28, 24, 35, 32],
'City': ['New York', 'Paris', 'Berlin', 'London']
}
df = pd.DataFrame(data)
print(df)
Output:
Name Age City
0 John 28 New York
1 Anna 24 Paris
2 Peter 35 Berlin
3 Linda 32 London
Example 2: Reading Data from a CSV File
Pandas make it easy to read data from external sources, such as CSV files, and perform operations on it.
import pandas as pd
# Reading a CSV file into a DataFrame
df = pd.read_csv('data.csv')
# Display the first 5 rows
print(df.head())
The `read_csv()` function loads the data from a CSV file into a DataFrame. You can then use various DataFrame operations to analyze and manipulate this data.
Example 3: Selecting Data
Pandas allows you to select rows and columns from a DataFrame efficiently using labels, indices, or conditions.
import pandas as pd
# Creating a simple DataFrame
data = {'Name': ['John', 'Anna', 'Peter', 'Linda'], 'Age': [28, 24, 35, 32], 'City': ['New York', 'Paris', 'Berlin', 'London']}
df = pd.DataFrame(data)
# Select a single column
print(df['Name'])
# Select multiple columns
print(df[['Name', 'City']])
# Select rows based on a condition
print(df[df['Age'] > 30])
Output:
0 John
1 Anna
2 Peter
3 Linda
Name: Name, dtype: object
Name City
0 John New York
1 Anna Paris
2 Peter Berlin
3 Linda London
Name Age City
2 Peter 35 Berlin
3 Linda 32 London
Example 4: Adding and Modifying Columns
You can easily add new columns to a DataFrame or modify existing ones:
import pandas as pd
# Creating a DataFrame
data = {'Name': ['John', 'Anna', 'Peter', 'Linda'], 'Age': [28, 24, 35, 32]}
df = pd.DataFrame(data)
# Adding a new column
df['Country'] = ['USA', 'France', 'Germany', 'UK']
# Modifying an existing column
df['Age'] = df['Age'] + 1
print(df)
Output:
Name Age Country
0 John 29 USA
1 Anna 25 France
2 Peter 36 Germany
3 Linda 33 UK
Example 5: Handling Missing Data
Pandas provides several functions to handle missing data in a DataFrame, such as detecting, removing, or filling null values.
import pandas as pd
import numpy as np
# Creating a DataFrame with missing values
data = {'Name': ['John', 'Anna', 'Peter', np.nan], 'Age': [28, 24, np.nan, 32], 'City': ['New York', 'Paris', np.nan, 'London']}
df = pd.DataFrame(data)
# Detect missing values
print(df.isnull())
# Fill missing values with a specific value
df_filled = df.fillna('Unknown')
print(df_filled)
# Drop rows with missing values
df_dropped = df.dropna()
print(df_dropped)
Output:
Name Age City
0 False False False
1 False False False
2 False True True
3 True False False
Name Age City
0 John 28.0 New York
1 Anna 24.0 Paris
2 Peter NaN Unknown
3 Unknown 32.0 London
Name Age City
0 John 28.0 New York
1 Anna 24.0 Paris
Example 6: Grouping Data
Pandas allows you to group your data based on certain columns and perform aggregate functions on them:
import pandas as pd
# Creating a DataFrame
data = {'Name': ['John', 'Anna', 'Peter', 'Linda', 'John', 'Anna'],
'Sales': [250, 200, 340, 310, 180, 220],
'Year': [2021, 2021, 2021, 2021, 2022, 2022]}
df = pd.DataFrame(data)
# Group by 'Name' and calculate total sales per person
grouped = df.groupby('Name')['Sales'].sum()
print(grouped)
Output:
Name
Anna 420
John 430
Linda 310
Peter 340
Name: Sales, dtype: int64
Example 7: Merging and Joining DataFrames
Pandas makes it easy to combine multiple DataFrames using various methods such as merging, joining, and concatenating.
import pandas as pd
# Creating two DataFrames
df1 = pd.DataFrame({'Name': ['John', 'Anna', 'Peter'], 'Age': [28, 24, 35]})
df2 = pd.DataFrame({'Name': ['John', 'Anna', 'Peter'], 'Country': ['USA', 'France', 'Germany']})
# Merging DataFrames on 'Name' column
merged_df = pd.merge(df1, df2, on='Name')
print(merged_df)
Output:
Name Age Country
0 John 28 USA
1 Anna 24 France
2 Peter 35 Germany
Conclusion
Pandas is an essential library for anyone working with Python data. Its flexible data structures and powerful manipulation tools make it easier to clean, analyze, and visualize large datasets. Whether you're working with time series, tabular data, or complex records, Pandas allows you to handle them efficiently and with minimal code.
With Pandas, tasks like reading files, filtering data, grouping, and merging can be done in a few lines of code, making it a must-learn library for data analysts and data scientists. Start exploring Pandas today, and it will quickly become your go-to tool for data analysis in Python.
What is Matplotlib Library in Python?
Matplotlib is a comprehensive and versatile plotting library for Python. It allows users to create a wide variety of static, animated, and interactive visualizations. From simple line charts to complex multi-panel figures, Matplotlib is capable of producing publication-quality graphs in various formats such as PNG, PDF, and SVG.
Matplotlib is often used alongside libraries like *NumPy* and *pandas* to visualize data stored in arrays and data frames. One of its key strengths is its flexibility and ability to generate plots that can be customized extensively, from fonts and labels to colors and styles.
Key Features of Matplotlib:
1. Plot Variety: Supports line plots, bar charts, scatter plots, histograms, pie charts, and more.
2. Customization: Allows customization of every part of a figure, from the size of the figure to the colors and labels used in the plot.
3. Integration: Works well with NumPy, pandas, and other libraries to provide a seamless data visualization experience.
4. Multiple Outputs: Supports multiple output formats, including interactive visualizations within Jupyter Notebooks.
5. Interactive Figures: Matplotlib enables users to zoom, pan, and save figures interactively in supported environments.
Getting Started with Matplotlib
You can install Matplotlib using `pip`:
pip install matplotlib
Once installed, import it using the following command:
import matplotlib.pyplot as plt
The `pyplot` module in Matplotlib provides a convenient interface for creating basic plots. Let’s dive into some examples
Example 1: Creating a Simple Line Plot
A line plot is one of the simplest types of plots that shows data as a continuous line.
import matplotlib.pyplot as plt
# Data
x = [0, 1, 2, 3, 4, 5]
y = [0, 1, 4, 9, 16, 25]
# Create a line plot
plt.plot(x, y)
# Adding labels and title
plt.xlabel('X-axis')
plt.ylabel('Y-axis')
plt.title('Simple Line Plot')
# Display the plot
plt.show()
Output:
A line graph is displayed, showing a curve representing the values of `y` as a function of `x`.
Example 2: Creating a Bar Chart
A bar chart is useful when you want to compare discrete categories or values.
import matplotlib.pyplot as plt
# Data
categories = ['A', 'B', 'C', 'D']
values = [5, 7, 3, 8]
# Create a bar chart
plt.bar(categories, values)
# Adding labels and title
plt.xlabel('Categories')
plt.ylabel('Values')
plt.title('Bar Chart Example')
# Display the plot
plt.show()
Output:
A bar chart is displayed with four bars representing the values for categories A, B, C, and D.
Example 3: Creating a Scatter Plot
A scatter plot is used to visualize the relationship between two continuous variables.
import matplotlib.pyplot as plt
# Data
x = [1, 2, 3, 4, 5]
y = [5, 3, 9, 6, 1]
# Create a scatter plot
plt.scatter(x, y)
# Adding labels and title
plt.xlabel('X-axis')
plt.ylabel('Y-axis')
plt.title('Scatter Plot Example')
# Display the plot
plt.show()
Output:
A scatter plot is displayed with points scattered at the positions defined by the `x` and `y` values.
Example 4: Creating a Histogram
A histogram is a useful plot for showing the distribution of a dataset.
import matplotlib.pyplot as plt
import numpy as np
# Generating random data
data = np.random.randn(1000)
# Create a histogram
plt.hist(data, bins=30, edgecolor='black')
# Adding labels and title
plt.xlabel('Value')
plt.ylabel('Frequency')
plt.title('Histogram Example')
# Display the plot
plt.show()
Output:
A histogram with 30 bins is displayed, showing the frequency distribution of the random data.
Example 5: Customizing Plots
Matplotlib allows you to customize every aspect of the plot. Here's how to change the color and style of the line, and add gridlines:
import matplotlib.pyplot as plt
# Data
x = [0, 1, 2, 3, 4, 5]
y = [0, 1, 4, 9, 16, 25]
# Create a customized line plot
plt.plot(x, y, color='green', linestyle='--', marker='o', markersize=8)
# Adding labels, title, and grid
plt.xlabel('X-axis')
plt.ylabel('Y-axis')
plt.title('Customized Line Plot')
plt.grid(True)
# Display the plot
plt.show()
Output:
A customized plot is displayed with a green dashed line, circular markers, and a grid.
Example 6: Subplots
Subplots are used to display multiple plots in a single figure.
import matplotlib.pyplot as plt
# Data
x = [1, 2, 3, 4, 5]
y1 = [1, 4, 9, 16, 25]
y2 = [25, 16, 9, 4, 1]
# Create a figure with two subplots (1 row, 2 columns)
plt.figure(figsize=(10, 4))
# First subplot (line plot)
plt.subplot(1, 2, 1)
plt.plot(x, y1, color='blue')
plt.title('Line Plot')
# Second subplot (bar plot)
plt.subplot(1, 2, 2)
plt.bar(x, y2, color='orange')
plt.title('Bar Chart')
# Display the figure with subplots
plt.tight_layout()
plt.show()
Output:
A figure with two subplots is displayed: a line plot on the left and a bar chart on the right.
Conclusion
Matplotlib is a powerful library for data visualization in Python, offering a wide range of plot types and customization options. Whether you're creating simple line plots or complex multi-panel figures, Matplotlib can help you visualize your data in a clear and effective manner. It integrates seamlessly with other scientific libraries like NumPy and pandas, making it an essential tool for data scientists, engineers, and anyone working with data in Python.
By learning how to use Matplotlib, you can enhance your ability to communicate insights through compelling visual representations of your data.
What is SciPy Library in Python?
SciPy is an open-source library that builds on the capabilities of NumPy and provides a collection of efficient numerical routines for scientific and technical computing in Python. It is a powerful tool for performing complex mathematical operations such as optimization, integration, interpolation, linear algebra, and statistics. SciPy is designed to work seamlessly with NumPy arrays and allows for easy manipulation of large datasets in various fields like machine learning, data science, and engineering.
Key Features of SciPy:
1. Integration with NumPy: SciPy extends NumPy’s functionality by adding higher-level mathematical operations, making it a go-to tool for scientists and engineers.
2. Optimization: Provides several optimization algorithms, including constrained and unconstrained optimization.
3. Integration: Performs both definite and indefinite integrals of functions and data points.
4. Linear Algebra: Offers operations like matrix decompositions, inverse matrices, eigenvalues, and more.
5. Signal Processing: Supports filtering, spectral analysis, and more for signal and image processing.
6. Statistical Functions: Provides distributions, tests, and descriptive statistics functions to analyze data.
7. Scientific Computing: Includes modules for Fourier transforms, interpolation, ODE solvers, and more.
Installing SciPy
To install SciPy, you can use the following command:
pip install scipy
Once installed, you can import it in your Python code:
import scipy
Let’s now explore an example that demonstrates how to use SciPy for solving a practical problem.
Example: Solving an Optimization Problem using SciPy
Optimization problems involve finding the best solution (e.g., maximum or minimum) from a set of possible solutions. SciPy provides the `optimize` module, which contains several functions for solving optimization problems.
Let’s say we want to minimize the function `f(x) = x^2 + 5x + 6`.
### Code:
import numpy as np
from scipy.optimize import minimize
# Define the function to minimize
def func(x):
return x**2 + 5*x + 6
# Initial guess (starting point for optimization)
x0 = 0.0
# Perform the minimization
result = minimize(func, x0)
# Output the result
print("Minimum value found at x =", result.x[0])
print("Minimum value of the function is =", result.fun)
Explanation:
1. Defining the Function: The function `func(x)` represents the mathematical function `f(x) = x^2 + 5x + 6`, which we want to minimize.
2. Initial Guess (`x0`): Optimization algorithms require an initial guess, which serves as the starting point. In this case, we start with `x0 = 0.0`.
3. Minimization (`minimize`): The `minimize` function from `scipy.optimize` is used to perform the minimization of `func(x)`. It returns the point `x` where the minimum occurs and the minimum value of the function.
4. Result: The `result.x` gives the value of `x` where the minimum is achieved, and `result.fun` gives the corresponding minimum function value.
Output:
Minimum value found at x = -2.5
Minimum value of the function is = -0.25
Conclusion
SciPy is an essential library for anyone working in scientific computing, as it provides a vast range of numerical algorithms to perform complex operations efficiently. It extends NumPy’s capabilities and adds specialized modules for optimization, integration, signal processing, linear algebra, and statistics. Whether you are solving optimization problems or performing statistical analysis, SciPy is a valuable tool for handling computational tasks in Python.
By using SciPy, you can perform high-level scientific computations with just a few lines of code, making your work faster, more accurate, and easier to manage.
What is Scikit-Learn and Statsmodels Library in Python?
In the realm of machine learning and statistical analysis, two powerful Python libraries are commonly used: Scikit-Learn and Statsmodels. Both libraries are essential tools for data analysis, modeling, and interpretation, though they cater to slightly different needs and workflows.
Scikit-Learn
Scikit-Learn is one of the most widely used Python libraries for machine learning. It provides simple and efficient tools for data mining and data analysis, and it is built on top of other libraries like NumPy, SciPy, and matplotlib. Scikit-Learn is particularly useful for implementing machine learning algorithms like classification, regression, clustering, and dimensionality reduction.
Key Features of Scikit-Learn:
1. Machine Learning Algorithms: Offers implementations of popular algorithms such as linear regression, decision trees, support vector machines, k-nearest neighbors, and many more.
2. Preprocessing Tools: Provides utilities for data preprocessing, such as scaling, encoding, and splitting datasets.
3. Model Selection: Supports techniques like cross-validation, grid search, and hyperparameter tuning.
4. Pipelines: Allows you to chain multiple steps of a workflow (e.g., preprocessing + modeling) for convenience and reproducibility.
Statsmodels
Statsmodels is another Python library focused more on the statistical side of modeling. It provides classes and functions for estimating and testing different statistical models, particularly for linear regression, generalized linear models (GLMs), time series analysis, and more. Statsmodels is ideal when you need detailed statistical information such as p-values, confidence intervals, and hypothesis tests, which are not the focus of Scikit-Learn.
Key Features of Statsmodels:
1. Detailed Statistical Output: Provides detailed output for statistical models, including parameter estimates, confidence intervals, p-values, and diagnostic tools.
2. Time Series Analysis: Offers built-in support for autoregressive models, moving averages, and ARIMA models.
3. Linear and Generalized Linear Models (GLMs): Supports various types of regression, from simple linear to logistic and Poisson regression.
4. Statistical Tests: Allows running hypothesis tests like t-tests, ANOVA, and goodness-of-fit tests.
Differences Between Scikit-Learn and Statsmodels
- Machine Learning vs. Statistics: Scikit-Learn is more focused on machine learning workflows, emphasizing prediction and performance. Statsmodels, on the other hand, emphasizes statistical models and hypothesis testing, providing more detailed outputs about the relationships between variables.
- Model Output: Scikit-Learn provides prediction accuracy and cross-validation scores, while Statsmodels provides more in-depth statistics like standard errors, p-values, and R-squared values.
Example: Linear Regression Using Scikit-Learn and Statsmodels
Let’s look at an example where we implement linear regression using both libraries.
Dataset:
We’ll use a simple dataset where we want to predict a dependent variable `Y` based on an independent variable `X`.
Example Code: Linear Regression with Scikit-Learn
# Importing necessary libraries
import numpy as np
from sklearn.linear_model import LinearRegression
import matplotlib.pyplot as plt
# Data (X and Y)
X = np.array([1, 2, 3, 4, 5]).reshape(-1, 1)
Y = np.array([1, 2, 4, 3, 5])
# Initialize and fit the model
model = LinearRegression()
model.fit(X, Y)
# Predicting values
Y_pred = model.predict(X)
# Display the coefficients
print("Slope (Coefficient):", model.coef_[0])
print("Intercept:", model.intercept_)
# Plotting the data and the regression line
plt.scatter(X, Y, color='blue')
plt.plot(X, Y_pred, color='red')
plt.title("Linear Regression using Scikit-Learn")
plt.xlabel("X")
plt.ylabel("Y")
plt.show()
Output:
- Slope (Coefficient): The coefficient of the independent variable `X`.
- Intercept: The value of `Y` when `X = 0`.
The plot will show a scatter plot of the data points and a red line representing the linear regression fit.
Example Code: Linear Regression with Statsmodels
import numpy as np
import statsmodels.api as sm
# Data (X and Y)
X = np.array([1, 2, 3, 4, 5])
Y = np.array([1, 2, 4, 3, 5])
# Adding a constant (intercept term) to X
X = sm.add_constant(X)
# Building the model
model = sm.OLS(Y, X)
results = model.fit()
# Displaying the summary of the model
print(results.summary())
Output:
This code will provide a full statistical summary of the linear regression model, including:
- Coefficients (Slope and Intercept): The estimated parameters of the model.
- P-values: The probability that the coefficient is statistically significant.
- R-squared value: The proportion of variance in the dependent variable that is predictable from the independent variable.
- Standard Errors: Estimates of the variability of the coefficients.
Conclusion
Both Scikit-Learn and Statsmodels are essential libraries in Python’s data science toolkit, but they serve different purposes.
- Scikit-Learn is the go-to library for machine learning algorithms and workflows where prediction accuracy is the focus.
- Statsmodels is used when a deeper understanding of statistical relationships and model diagnostics is required.
Whether you're building a predictive model or performing a statistical analysis, knowing when and how to use these libraries is key to effective data science. By combining the strengths of both, you can leverage the power of machine learning while ensuring a solid statistical foundation for your models.
Comments
Post a Comment