What is a Data Structure in Python?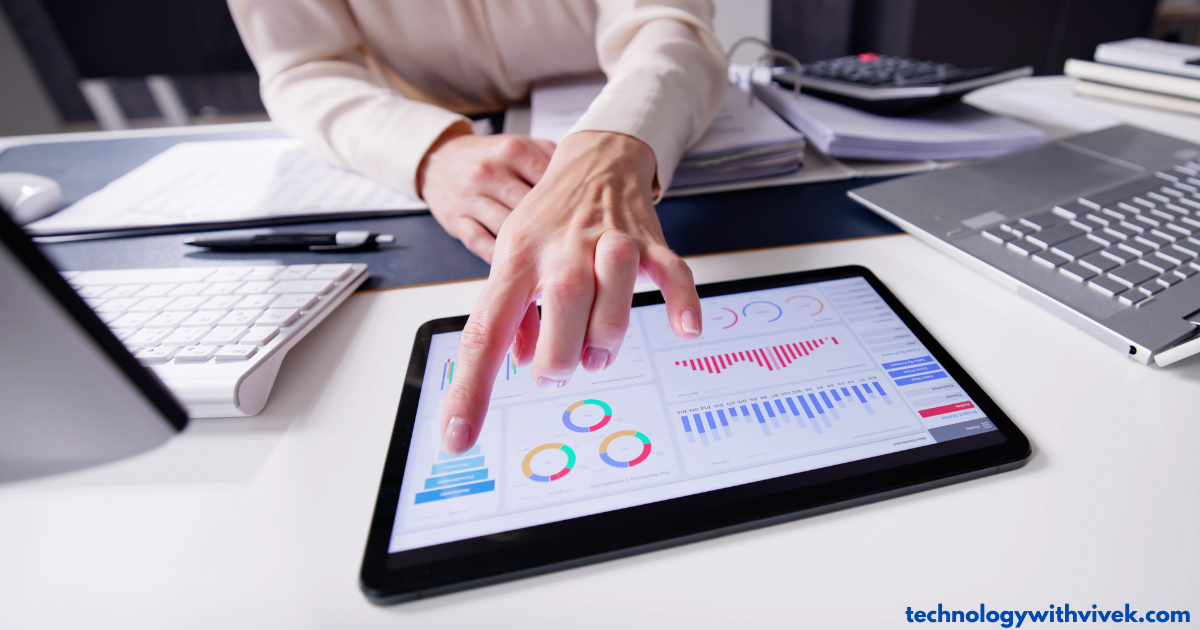
In computer science, a data structure organizes and stores data to be accessed and modified efficiently. In Python, data structures are fundamental constructs that allow you to store data collections, retrieve and manipulate data, and implement various algorithms.
One of the most commonly used data structures in Python is the list. Python's list is a mutable, ordered collection that allows elements of different types. Lists are highly versatile, and Python provides several built-in methods to work with lists. This article explores how to use common list operations in Python.
Creating a List in Python
You can create a list in Python by placing elements inside square brackets `[]`. Here’s an example of creating a simple list:
# Creating a list of numbers
my_list = [1, 2, 3, 4, 5]
### Common List Operations in Python
1. append()
Adds a single element to the end of the list.
my_list.append(6)
print(my_list) # Output: [1, 2, 3, 4, 5, 6]
2. extend()
Extends the list by appending all the elements from an iterable (such as another list).
my_list.extend([7, 8])
print(my_list) # Output: [1, 2, 3, 4, 5, 6, 7, 8]
3. insert()
Inserts an element at a specific position in the list. The first argument is the index where you want to insert the element.
my_list.insert(2, 'a') # Insert 'a' at index 2
print(my_list) # Output: [1, 2, 'a', 3, 4, 5, 6, 7, 8]
4. index()
Returns the index of the first occurrence of the specified value.
index_of_3 = my_list.index(3)
print(index_of_3) # Output: 3
5. remove()
Removes the first occurrence of the specified element from the list.
my_list.remove('a')
print(my_list) # Output: [1, 2, 3, 4, 5, 6, 7, 8]
6. pop()
Removes the element at the specified position and returns it. If no index is specified, it removes and returns the last element.
last_item = my_list.pop() # Removes the last element
print(last_item) # Output: 8
print(my_list) # Output: [1, 2, 3, 4, 5, 6, 7]
7. count()
Returns the number of occurrences of a specified element in the list.
count_of_3 = my_list.count(3)
8. sort()
Sorts the list in ascending order by default. You can use the `reverse=True` argument to sort in descending order.
my_list.sort()
print(my_list) # Output: [1, 2, 3, 4, 5, 6, 7]
my_list.sort(reverse=True)
print(my_list) # Output: [7, 6, 5, 4, 3, 2, 1]
9. reverse()
Reverses the order of the elements in the list.
my_list.reverse()
print(my_list) # Output: [1, 2, 3, 4, 5, 6, 7]
Slicing a List
Slicing allows you to access a range of elements from the list using the syntax `list[start:end]`. The `start` index is inclusive, while the `end` index is exclusive.
# Slicing the first three elements
slice_of_list = my_list[0:3]
print(slice_of_list) # Output: [1, 2, 3]
You can also use negative indexing to slice from the end of the list:
# Last three elements
last_three = my_list[-3:]
print(last_three) # Output: [5, 6, 7]
List Comprehension
List comprehension provides a concise way to create lists based on existing lists. It allows you to perform operations or filter elements while creating the list.
# Creating a list of squares of numbers from 1 to 5
squares = [x**2 for x in range(1, 6)]
print(squares) # Output: [1, 4, 9, 16, 25]
### Copying a List
There are different ways to copy a list in Python:
1. Shallow Copy
A shallow copy creates a new list, but it does not recursively copy the objects within the original list. If the list contains objects, only the references to those objects are copied.
shallow_copy = my_list.copy() # or shallow_copy = list(my_list)
2. Deep Copy
A deep copy creates a new list, and recursively copies all objects found within the original list. This ensures that all elements are entirely copied, not just referenced.
import copy
deep_copy = copy.deepcopy(my_list)
Conclusion
In Python, lists are incredibly versatile and powerful data structures that can be used in various ways. From basic operations like adding and removing elements to more advanced concepts like slicing and copying, lists provide a wide range of functionality. Understanding how to effectively use these methods and operations can make your code more efficient and easier to manage.
By mastering these techniques, you can handle data with ease, making Python's list an invaluable tool in your coding toolkit!
Comments
Post a Comment