Program: Data Visualization using Matplotlib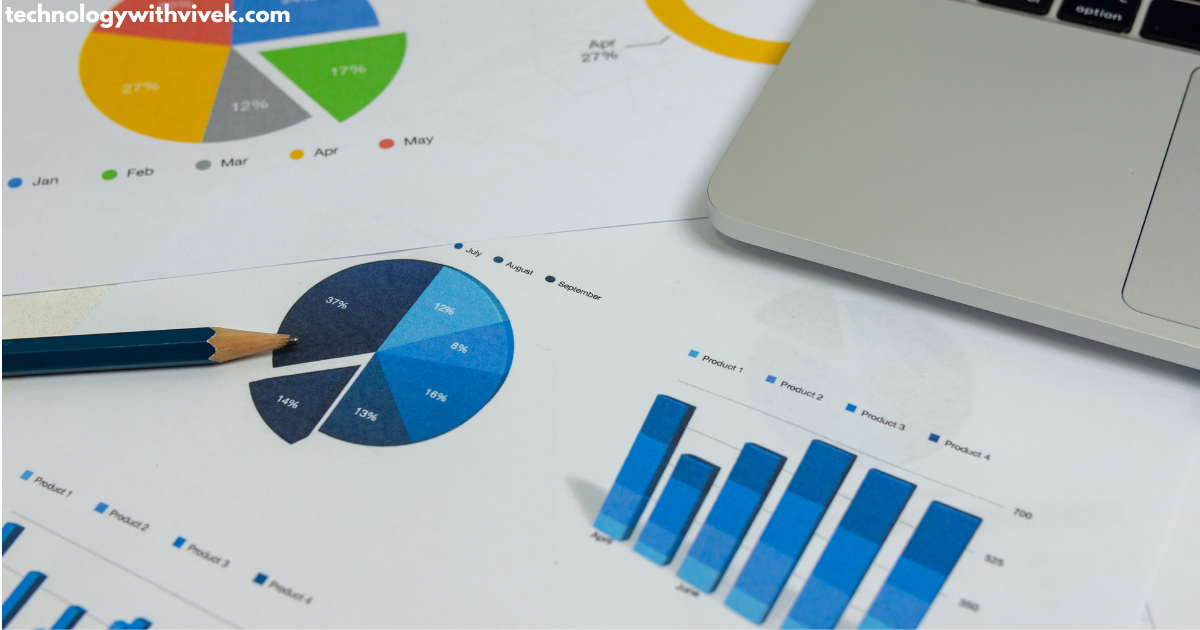
This program demonstrates how to create multiple types of plots using Matplotlib for visualizing a dataset. It includes line plots, bar charts, scatter plots, and pie charts.
Scenario: Sales Data Analysis
We have sales data for different regions over a year. The goal is to visualize trends, distributions, and comparisons.
Code Implementation
import matplotlib.pyplot as plt
import numpy as np
# Sample Data
months = ['Jan', 'Feb', 'Mar', 'Apr', 'May', 'Jun', 'Jul', 'Aug', 'Sep', 'Oct', 'Nov', 'Dec']
region_a_sales = [300, 400, 350, 500, 600, 700, 750, 800, 650, 550, 450, 400]
region_b_sales = [200, 300, 250, 450, 500, 650, 700, 750, 600, 500, 400, 300]
region_c_sales = [100, 150, 200, 300, 400, 500, 550, 600, 500, 400, 300, 200]
regions = ['Region A', 'Region B', 'Region C']
# --- 1. Line Plot: Monthly Sales Trends ---
plt.figure(figsize=(10, 6))
plt.plot(months, region_a_sales, label='Region A', marker='o')
plt.plot(months, region_b_sales, label='Region B', marker='s')
plt.plot(months, region_c_sales, label='Region C', marker='^')
plt.title('Monthly Sales Trends')
plt.xlabel('Months')
plt.ylabel('Sales ($)')
plt.legend()
plt.grid(True)
plt.show()
# --- 2. Bar Chart: Total Sales Comparison ---
total_sales = [sum(region_a_sales), sum(region_b_sales), sum(region_c_sales)]
plt.figure(figsize=(8, 5))
plt.bar(regions, total_sales, color=['blue', 'orange', 'green'])
plt.title('Total Sales by Region')
plt.xlabel('Regions')
plt.ylabel('Total Sales ($)')
plt.show()
# --- 3. Scatter Plot: Region A vs. Region B Sales ---
plt.figure(figsize=(8, 5))
plt.scatter(region_a_sales, region_b_sales, color='purple')
plt.title('Region A vs. Region B Sales')
plt.xlabel('Region A Sales ($)')
plt.ylabel('Region B Sales ($)')
plt.grid(True)
plt.show()
# --- 4. Pie Chart: Sales Distribution ---
plt.figure(figsize=(8, 8))
plt.pie(total_sales, labels=regions, autopct='%1.1f%%', startangle=140, colors=['skyblue', 'orange', 'lightgreen'])
plt.title('Sales Distribution by Region')
plt.show()
# --- 5. Combined Plot: Highlighting Trends and Totals ---
plt.figure(figsize=(10, 6))
width = 0.25 # Bar width
x = np.arange(len(months)) # X positions for bars
# Bar plots for monthly sales
plt.bar(x - width, region_a_sales, width, label='Region A', alpha=0.8, color='blue')
plt.bar(x, region_b_sales, width, label='Region B', alpha=0.8, color='orange')
plt.bar(x + width, region_c_sales, width, label='Region C', alpha=0.8, color='green')
# Line plot overlay for totals
plt.plot(months, np.array(region_a_sales) + np.array(region_b_sales) + np.array(region_c_sales),
label='Total Sales', color='red', marker='o', linewidth=2)
plt.title('Monthly Sales by Region with Total Trend')
plt.xlabel('Months')
plt.ylabel('Sales ($)')
plt.xticks(x, months)
plt.legend()
plt.show()
Explanation of Code
1. Line Plot:
- Visualizes monthly sales trends for all regions with distinct markers and labels.
- Helps identify peaks and troughs in sales.
2. Bar Chart:
- Compares total sales across regions.
- Useful for highlighting differences in overall performance.
3. Scatter Plot:
- Examines the relationship between sales of Region A and Region B.
- Highlights potential correlations.
4. Pie Chart:
- Shows the percentage distribution of total sales across regions.
- Useful for understanding regional contributions.
5. Combined Plot:
- Combines bar charts for monthly regional sales with a line plot for total sales.
- Provides a comprehensive view of sales trends and totals.
Key Features of Matplotlib Used
Customization:
- Titles, axis labels, legends, and gridlines.
Styling:
- Different colors, markers, and line styles.
Multiple Plot Types:
- Line, bar, scatter, pie, and combined plots.
Annotations:
- Labels like `autopct` in pie charts and legends in all plots.
Sample Visuals
1. Line Plot:
Shows how sales fluctuate month by month for each region.
2. Bar Chart:
Highlights which region performed the best in total sales.
3. Scatter Plot:
Helps in identifying relationships between two regions' sales.
4. Pie Chart:
Demonstrates the proportion of sales from each region.
5. Combined Plot:
Offers a consolidated view for detailed analysis.
Program Extension
- Add interactivity with Plotly for dynamic visualizations.
- Use real-world sales datasets from CSV or databases.
- Save plots as images using `plt.savefig('filename.png')`.
This program serves as a foundational tool for analyzing and presenting data visually using Matplotlib.
Comments
Post a Comment